How to structure data for D3.js
In this section, you will understand how to structure data for better use of D3.js.
We will cover where we have been, how D3.js thinks of selections, the basics of JavaScript objects and how to best structure our data.
What we have seen thus far
Every example we have done with D3.js thus far has included a data set at the top of the example like this one:
This variable spaceCircles is a JavaScript Array.
An Array is an enumerated list of variables.
This means that each element has an index number attached to it, starting with the number 0.
In the spaceCircles case if we type the following into the JavaScript Console to get the first element (note, JavaScript arrays are 0-indexed):
we'll get:
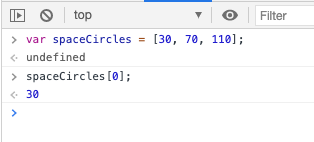
To get the second JavaScript array element, we type:
which gives us:

To the the third, and final, JavaScript array element, we type:
which gives us:
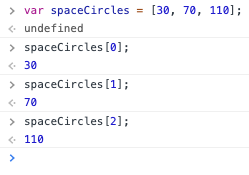
JavaScript Arrays can hold any type of information you want to put into them.
You can have arrays of numbers, objects, strings, arrays, HTML Elements, DOM Elements, etc.
D3 Data Operator Can Take In And Use JavaScript Arrays
If you recall the Use Data Bound To DOM Elements With D3.js section, we covered Variables Available inside the D3.js Data functionality.
One of those variables was the index, or i. This variable refers to the Array index of the selection that D3.js is referencing.
Which is why the following code worked:
and gave us this result:
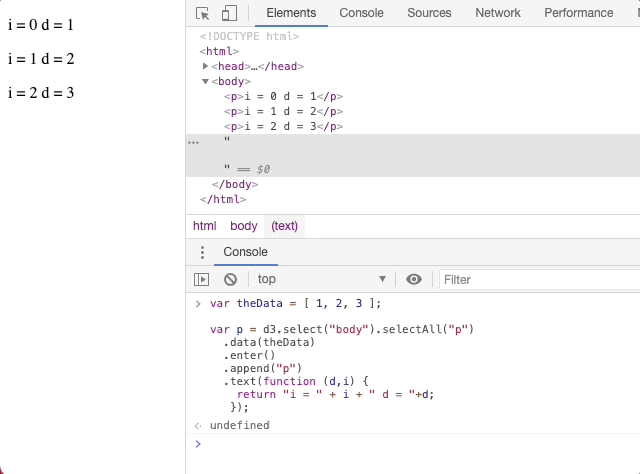
So you can see that the theData JavaScript variable is an array and the D3 Data operator ingested the data and then used it to generate HTML Paragraph DOM elements that are data-driven.
Which means that it's important to make sure that the .data() operator receives an array of data, regardless of what is inside of the array.
Loading External Data Resources
D3.js has built in functionality to load in the following types of external resources:
- an XMLHttpRequest
- a text file
- a JSON blob
- an HTML document fragment
- an XML document fragment
- a comma-separated values (CSV) file
- a tab-separated values (TSV) file
Each of these resources will return data that D3.js can then use.
The only thing to pay attention to is that you want to make sure you construct an array out of the data.
So far we are manually inputing our data array and will continue to do so for a while longer.
Later sections will cover how load resources from a server.